An Introduction to Java Arrays
In. this post we introduce and define the array data structure in java, how to use it and what common operations can be performed using arrays.
What is an Array in Java?
An array is a fixed-length Java container object that contains other objects of the same type. An array’s length and the type of object it contains need to be defined upon creation. An array is iterable and objects can be accessed by index.
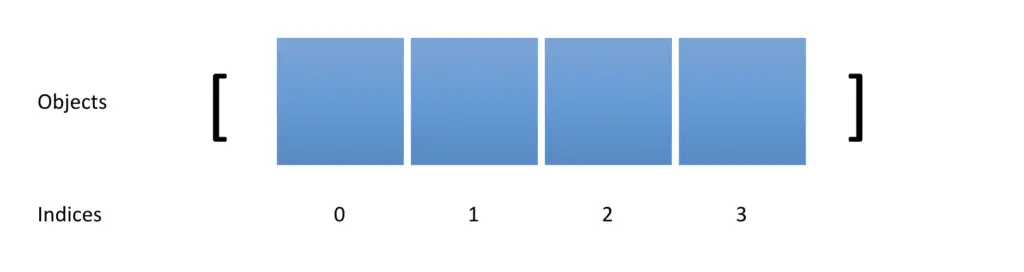
How to Initialize an Array in Java?
Initializing an array requires that we first declare a variable to which the array is assigned along with its type. Arrays are declared using the type of contained object followed by double brackets. Then we can specify the name we want to give to the newly created array object.
Here we are declaring a java string array, followed by a java int array (int stands for integer).
String[] stringArray; int[] intArray;
Now we can initialize the array using the new keyword and the number of elements the array will contain.
String[] stringArray = new String[10]; int[] intArray = new int[10];
How to Access and Assign Values in a Java Array?
We can access a single element in a Java array using the index of the element.
You can print array elements to the console using the Java system’s print function.
System.out.println(stringArray[0]); //null System.out.println(intArray[0]); //null
If you run this and print the output to the console, it will return null values. The reason is that although the arrays have been created, they don’t contain any values. They only contain placeholders for the specified length.
For every placeholder, we can assign a value of the specified type.
stringArray[0] = "Hello"; intArray[0] = 1;
If you access and print the array elements after assigning values, it should print the elements you assigned to the console.
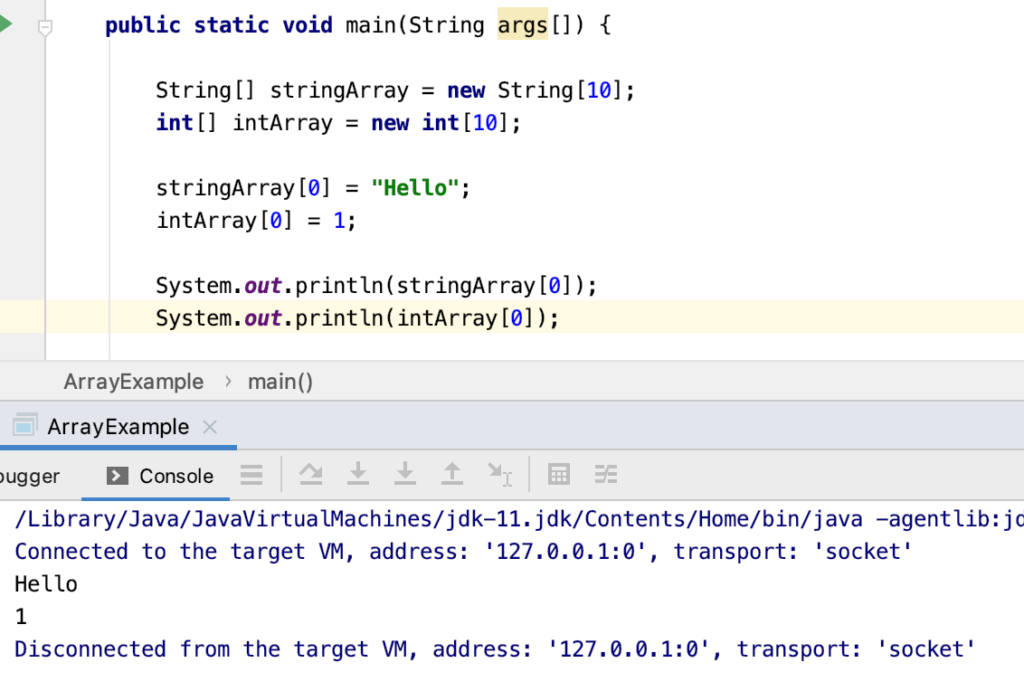
Assigning Values upon Array Creation
It is also possible to pre-fill the array with default values.
String[] stringArrayPrefilled = new String[] { "Hello", "Bye", };
When we initialize the array with values, we do not need to specify a length. Java automatically infers the length from the number of objects assigned.
The new keyword and the type specification are not necessary when creating the array. So we can write this in a more succint form:
String[] stringArrayPrefilled = { "Hello", "Bye", };
Creating a Java Array of Objects
In addition to primitive types such as strings and ints, you can also create arrays of your own custom objects.
public class ExampleObject { int number; String text; public ExampleObject(int number, String text){ this.number = number; this.text = text; } }
Next, we create an empty array of type ExampleObject, create an instance of that object and add it to the array.
ExampleObject[] objectArray = new ExampleObject[5]; ExampleObject object = new ExampleObject(1, "Hello"); objectArray[0] = object;
Or alternatively, we can pre-fill the array with the object.
ExampleObject[] objectArrayPrefilled = new ExampleObject[] { new ExampleObject(1, "Hello") };
Splitting a String into a Java Array
If you have a string that is separated by a certain symbol like a comma, you can also use regular expressions to split the string into an array.
String text = "H,e,l,l,o"; String[] stringArraySplit = text.split("\\,", -1);
How to Print an Array in Java
There are three main ways to print the entire array in Java: Converting to a String; iterating over the array, and printing the single elements; and using lambda expressions.
Convert the Array to a String
The Java Util package contains a function that enables us to print an array.
String[] array = new String[] {"Hello", "Bye"}; System.out.println(Arrays.toString(array));
Iterate through the Array
The next option is to iterate through the array and print every single element.
for (String s : stringArray) { System.out.println(s); }
Using Java Lambda Expressions
If you are using Java 8 or later, you can also use lambda expressions for printing objects. The lambda expression allows you to iterate through the array using the forEach method and simply passing the print function as an argument to the forEach function.
String[] array = new String[] {"Hello", "Bye"}; Arrays.stream(array).forEach(System.out::println);
Note that if you want to print custom objects, they need to have implemented a toString method so Java knows how to print them.
How to Find the Array Length in Java
We obtain the length of a Java array by calling the length attribute on the created array object. The array length in Java is predefined upon array creation and cannot be changed because arrays are fixed-length data structures.
String[] stringArray = new String[10]; int len = stringArray.length; System.out.println(len);
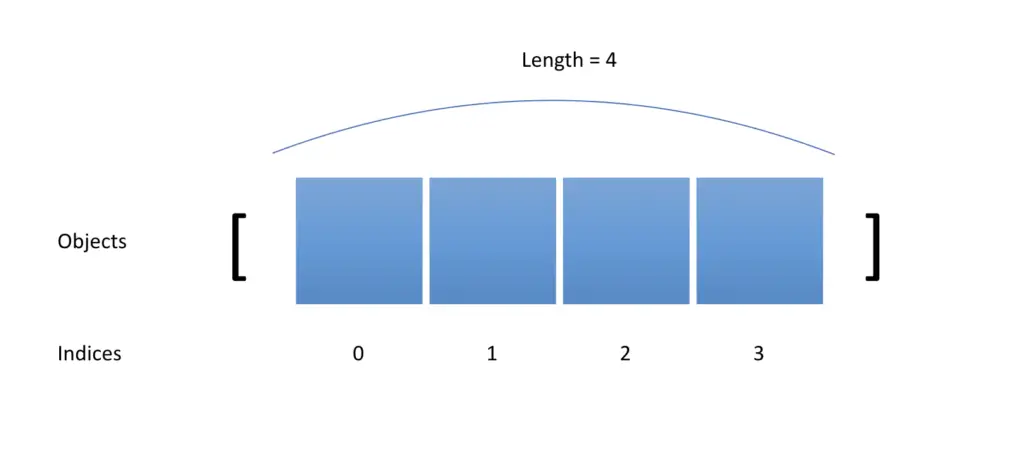
Adding and Removing Elements in a Java Array
Since arrays are fixed-length data structures, you cannot add or remove elements directly.
Add an Element to an Array in Java
If you want to add an item, you have to create a new array whose length is incremented by one compared to the original array.
String[] stringArrayPrefilled = new String[] { "Hello", "Bye", }; int l = stringArrayPrefilled.length; String newarr[] = new String[l + 1];
Then you copy the old array over using a for loop and assign the new element to the last index. The last index l is equivalent to the length of the old array since Java starts indexing from zero. For example, if an array contains 10 items, it has a length of 10 and indices ranging from 0 to 9.
for (int i = 0; i < l; i++) { newarr[i] = stringArrayPrefilled[i]; } newarr[l] = "Good Morning";
Printing this to the console reveals that the new array has three elements with “Good Morning” being the last.
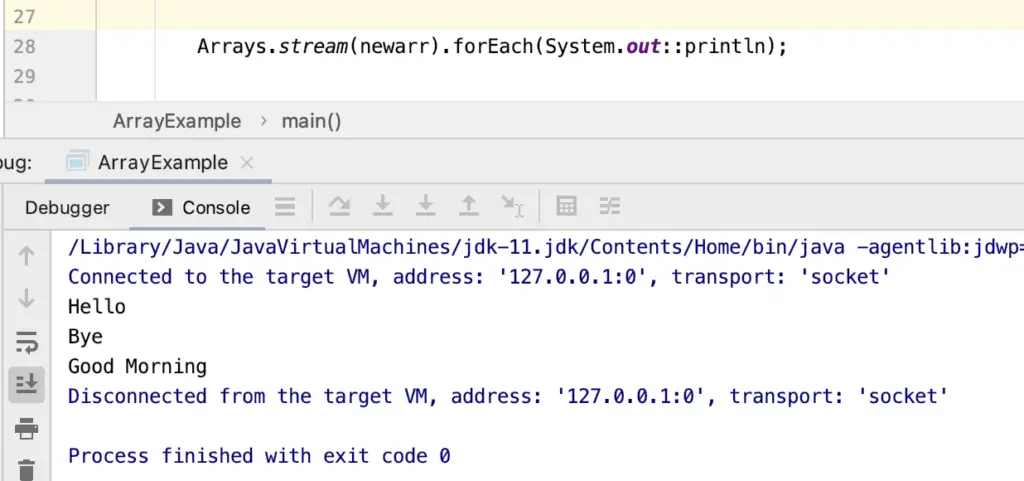
Remove an Element from an Array in Java
To remove an element from an array, we need to create a new empty array that is smaller than the first array by one element.
String[] stringArrayPrefilled = new String[] { "Hello", "Bye", "Good Morning" }; int l = stringArrayPrefilled.length; String newarr[] = new String[l - 1];
Next, we iterate through the old array up until the last index of the new array and copy values over.
for (int i = 0; i < newarr.length; i++) { newarr[i] = stringArrayPrefilled[i]; }
If we print the new array and its length, it displays the first two items of the original array and a length of two (compared to three in the original array).
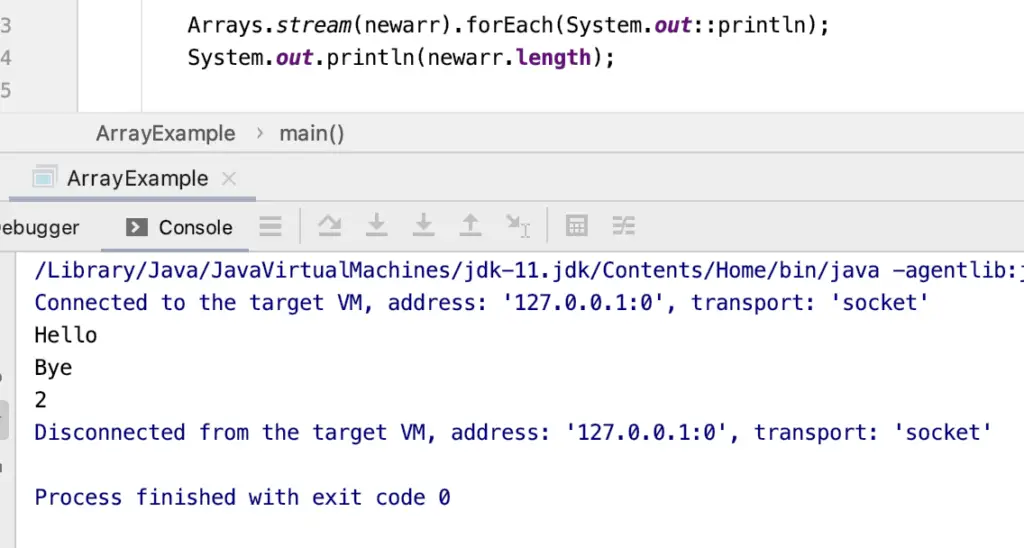
How to Sort an Array in Java
Unless you want to write your own implementation of a sorting algorithm, the most convenient way to sort an array in Java is the sort function from the java utils package. It sorts the array in place.
int[] intArrayPrefilled = new int[] { 4, 1, 2, 6, 5 }; Arrays.sort(intArrayPrefilled); #print the sorted array Arrays.stream(intArrayPrefilled).forEach(System.out::println);
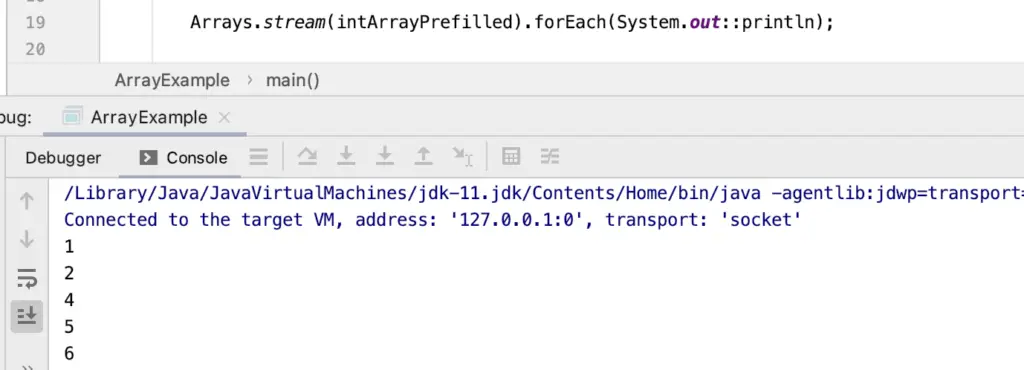
Array-List Conversion
Lists are more flexible and efficient when it comes to appending and removing values at the start or the end of an array. They are variable length data structures, which means their length is not fixed upon creation. A list is an abstract data type. The concrete implementation is the Java ArrayList.
Convert Java Array to a List
To convert an array to a list in Java, you can use the asList function from the Java Utils package. We take our initial array, pass it to the asList function and assign the result to a list of integers. Since the list is an abstract data type, we have to use the ArrayList when creating the concrete converted data type.
Integer[] intArrayPrefilled = {4, 1, 2, 6, 5}; List<Integer> intList = new ArrayList<Integer>(Arrays.asList(intArrayPrefilled));
Convert a List to an Array in Java
To convert a list to an array we can use the toArray method directly on the list. Since we cannot initialize an array with initial values directly, we use the asList method from the array package to create our list.
List<Integer> intList = Arrays.asList(4, 1, 2, 6, 5); Integer[] intArray = intList.toArray(new Integer[0]);
The argument “new Integer[0]” that we pass to the toArray method tells Java to treat this as an array of integers.