How to Learn Java: A Comprehensive Guide
Java is one of the most widely used programming languages in the world today. It powers applications ranging from enterprise software systems to Android apps. Learning it can open the door to plenty of well-paid job opportunities. People who have mastered the art of developing software in Java are hot commodities in the job market. So how do you learn it?
To learn Java, you need to understand software design fundamentals, including object-oriented design, data structures, and classical algorithms for searching and sorting. In addition, you should become familiar with good programming practices, and Java frameworks such as Spring and build tools such as Maven.
We’ll discuss in detail what you really need and how long it takes. But let’s start with the most immediate questions.
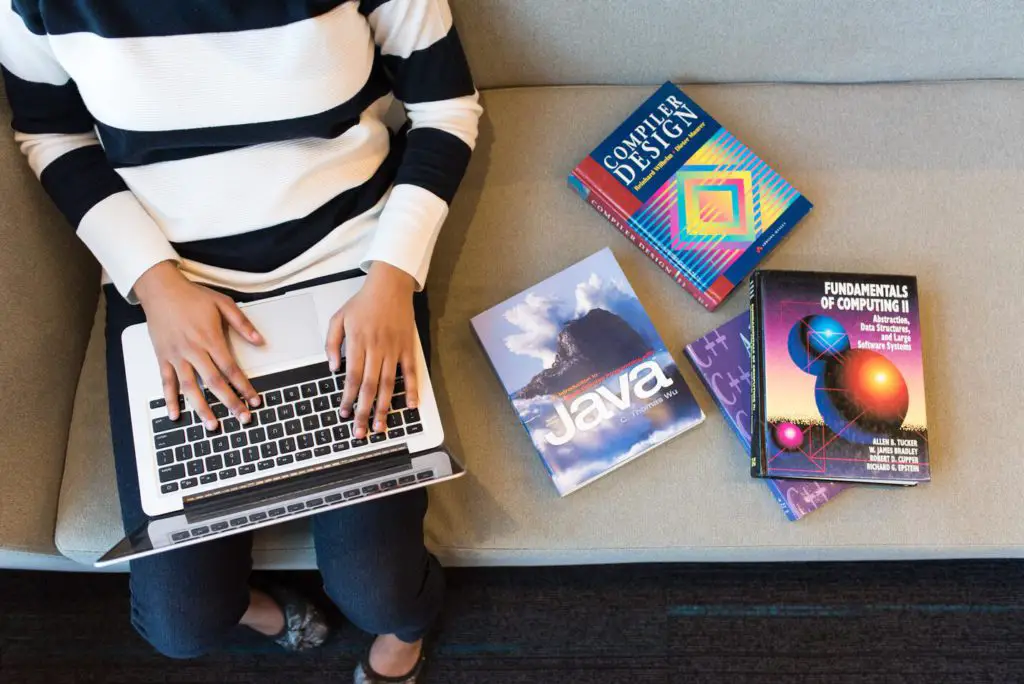
Disclosure: Some of the links in the following sections are affiliate links. This means I may earn a small commission at no additional cost to you if you decide to purchase. I could be an affiliate for many online education products. I’ve specifically chosen to partner with the providers of courses and books that I recommend based on my own experience.As an Amazon affiliate, I earn from qualifying purchases.By using my links you help me provide information on this blog for free.
How do I start learning Java
If you are self-studying, your best bet is to look for a solid online course on a platform like Coursera that teaches Java interactively through programming assignments. But it is also important that the course features good explanations of programming concepts. Otherwise, you’ll end up frustrated and confused.
If you haven’t done so already, I also recommend downloading an IDE and getting started with Java development on your own machine. The reason is that it gives you a much better insight into all the issues involved in setting up a programming environment before you can actually start coding. Most courses and books on software engineering fundamentals in Java usually have a section that includes setting up your development environment.
I highly recommend the “Object-Oriented Programming in Java Specialization” on Coursera.
It features great explanations and interesting programming assignments. By the end of the specialization, you should have a solid foundational grasp of software engineering in Java.
Once you have gone through a basic Java course on a learning platform, you should be familiar with basic concepts such as variables, data structures, classes, objects, loops, etc.
Next, try to build your own object-oriented pet project. Here is an example:
Design a parking lot that allows entry for 2-3 classes of vehicles, has different tickets and prices associated with each vehicle and how long they stay. Before letting a vehicle enter, the system needs to check whether there are still spaces available. After the vehicle has entered, the system has to update the number of available spaces. When a vehicle wants to leave, the system needs to calculate the correct ticket price, charge the customer, and update the number of available spaces.
If you can get a system like this to work, you are well on your way towards becoming a great Java developer.
Is it Easy to Learn Java?
Learning Java is not difficult if you have the right resources and the right guidance. Use interactive learning platforms to master the basics, then start building your own Java projects as soon as possible.
Some concepts such as classes, objects, abstraction, and inheritance can be hard to wrap your head around. This is especially true if you are new to object-oriented programming. If you have previous experience in procedural programming, don’t make the mistake of applying the same principles to an object-oriented language like Java. It is a different paradigm.
Can I teach myself Java?
Thanks to interactive learning platforms and high-quality online courses, learning Java on your own has become much easier in recent years. Previous generations of developers only had access to abstract books. Make use of these interactive tools. But also read some books to really deepen your understanding. For example, I’ve benefitted immensely from reading books like Robert Martin‘s “Clean Code” or Martin Fowler‘s “Refactoring” which teach fundamental principles and best practices of software engineering.
What do I have to Learn?
With the exception of the fundamentals and the practices, what you need to learn depends largely on your area of interest or the area where you want to work.
Java Programming Fundamentals
Software design fundamentals are really the foundation since Java is a language used predominantly for building software systems. You definitely need to be familiar with:
- Object-Oriented Programming including classes, objects, abstraction, encapsulation, inheritance, and polymorphism
- Data Structures such as arrays, queues, stacks, lists, and hashmaps.
- Operators such as loops
- Algorithms for searching and sorting (most algorithms have been implemented in packages, so being able to implement them yourself is not a priority. It really helps you become a better developer though because you learn a lot about the efficiency and performance of a program if you implement an algorithm yourself)
Those are the foundations that you build upon with everything else you do. There are lots of resources online for learning the basics. The fastest way to learn them are courses with an interactive coding component.
Practices
Testing and Test Driven Development
If you write production code or generally more than a few lines of code, I strongly encourage you to write tests. There are three types of tests:
- Unit Tests: Used to test single functions and methods
- Integration tests: Test the interactions between classes and modules
- System-level Tests: These tests treat the system as a black box, interact with it through external interfaces, and check whether it returns the expected values.
As a developer, you ideally write unit tests in parallel with the production code. The practice of test-driven development goes one step further by stipulating that you should write a test before you write a production method. The basic testing tool in Java is JUnit. Definitely check it out once you have learned the fundamentals.
Refactoring & Clean Code
Clean code stipulates that you should keep your codebase neat, organized, and flexible. Refactoring is intimately connected to clean code.
It is the practice of changing and cleaning your code without altering its functionality or how it interacts with other components of your system. This is a vital skill for any software engineer because it is impossible to plan what your code will look like once the system is finished. Instead, you should practice emergent design. As you write more code, the structure of the system will slowly emerge and become clearer. Often you will realize that the code you’ve written before doesn’t work well with your requirements and the current structure of your system. Sometimes the external requirements change, or you have just piled on so much code in one place that you have a hard time understanding it. Then you have to go back and change the code to fit your new requirements and make it generally cleaner and more expressive.
This is one of the main reasons why Java and other object-oriented languages are built around principles like abstraction and encapsulation. They enable you to keep your components and classes separated so that you can easily change their internal workings without affecting their external interactions. A good practice to make refactoring easier and maintain clean code is to define contracts between objects in the form of interfaces wherever possible. Then you can build the object however you like as long as it abides by the contract.
Clean code is a principle to live by as a software developer, while refactoring is a continuous practice that you should keep up from the start through the end of your project.
Agile Development
Agile development is a practice to keep your workflow and your codebase flexible so that you can react to changing requirements and conditions. You gather all the requirements you currently have and design a plan to implement them that fits into a specified timeframe called a sprint (often one or two weeks). All those features that cannot be implemented in that window go into a backlog. Within the timeframe, you and your collaborators work on implementing the features while regularly checking whether you adhere to the requirements and if they have changed.
After finishing a sprint, you reevaluate the requirements and your current software architecture. Based on your conclusions, you define the next work batch for another sprint.
This practice is usually superior compared to planning everything in advance because it allows you to maintain a high degree of flexibility and forces you to constantly keep the bigger picture in mind.
Testing, refactoring, and agile development are important components in every professional Java developer’s toolbox. Unfortunately, they are not as straightforward to learn as programming fundamentals. Instead, they are skills you need to build and nurture throughout your programming career. This is one of the main reasons why many employers are reluctant to take on a fresh graduate. They may be great programmers, but they lack the practices that make a great developer. Luckily for us, there is such a shortage of qualified developers that you should still be able to find an entry-level position as a decent programmer.
There are online courses on platforms like Coursera and Pluralsight, which teach you the fundamentals. But the real insights come from reading the code of great developers and reading books by programming legends such as Martin Fowler or Robert Martin.
Frameworks
What frameworks you need to learn depends on your area of specialization. Java is a language that is extremely popular with enterprise software development, an area that is usually well-funded. One of the most popular Java frameworks for enterprise application development is Spring. If you want a well-paid job in enterprise Java development, you should probably learn Spring. In addition, it is probably also a good idea to learn a framework for communicating with databases such as Hibernate.
Java is also the language that has originally been used to develop Android applications. If you learn Java to build Android apps, you won’t need to know Spring. But I would definitely encourage you to learn Kotlin, a higher-level language built on top of the Java virtual machine. Google aims to replace Java as the standard language for Android development with Kotlin. Learning Kotlin should be easy after you’ve mastered Java.
There are dozens of other Java frameworks around for building desktop and web applications. You need to do your own research on which ones are necessary for your area of expertise and interest.
To learn specific frameworks, you’ll find online courses on platforms like Udemy and Pluralsight or books on Amazon. Just search these platforms for the title of the framework.
Tools
Build tools such as Maven and Gradle should definitely be part of your toolbox if you want to build and deploy software. They allow you to package and deploy your code, as well as manage dependencies such as external packages which add functionality to your system.
If you have worked in a software development role before, you are probably familiar with git. Git is a version control system that makes it easy to roll back unwanted changes and to merge your code with that of other developers. Anyone who wants to develop software collaboratively with others should definitely know git.
If you write any program that is longer than a few lines of code, you should make it a habit to write tests. JUnit is the default unit testing tool that comes with most IDEs. You should definitely become familiar with it and with testing Java code in general.
As with frameworks, there are many tools which you can do your own research on.
How long does it take to learn Java?
You can learn the fundamentals of Java programming within 2 – 3 weeks if you commit to an hour of learning every day. But solidifying these concepts and becoming a decent Java developer who can write production code will take several months of practice.
Once you are proficient in the programming fundamentals of Java, you can learn most tools and frameworks within a couple of days. Again, solidifying your knowledge takes longer.
Practices such as clean code, refactoring, testing, and agile development are something you should work on improving throughout your entire career as a developer.