Dictionaries, Tuples, and Sets in Python
In this post, we introduce three foundational data structures in Python: the dictionary, the tuple, and the set.
What is a Dictionary in Python?
A dictionary is a data structure that stores items using key-value pairs. Each entry needs to have a unique key. Entries are ordered and can be changed. Dictionaries come inbuilt with Python and are created using curly brackets.
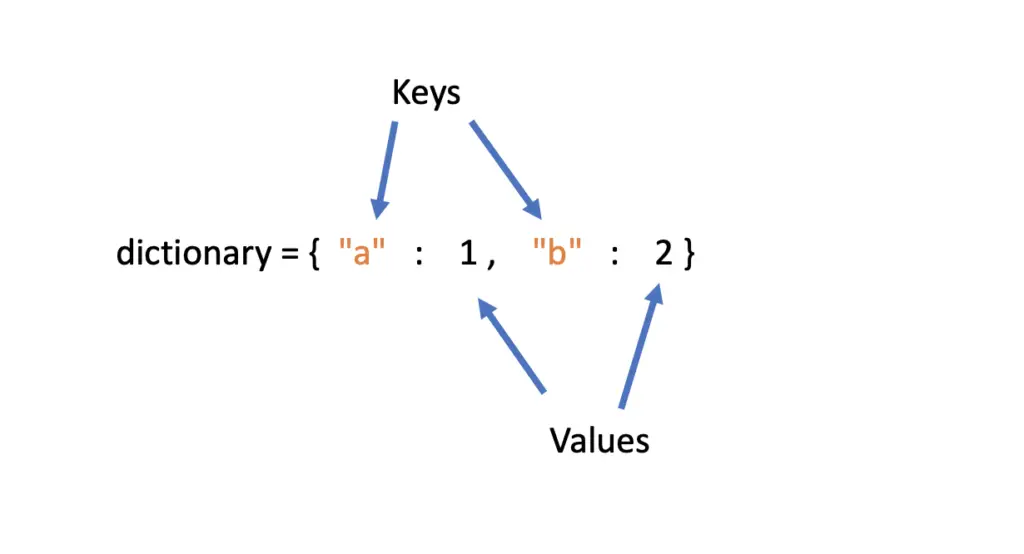
How Do You Create a Dictionary in Python
You create a Python dictionary using curly brackets or the “dict” function. You can either pre-fill the dictionary upon creation or add items after the dictionary has been initialized.
# Initializing a dictionary with values dictionary = {"a" : 1 , "b" : 2} #Creating an empty dictionary dictionary = {} #Creating an empty dictionary with the dict function dictionary = dict()
How to Add to A Dictionary in Python
You add values to a dictionary by indexing a new key and assigning a value to it.
dictionary = {} dictionary["c"] = 3 print(dictionary) # returns {'c': 3}
How to Sort a Dictionary in Python?
The very purpose of a dictionary is to find specific values by their respective keys. Accordingly, the order of items in a dictionary shouldn’t matter in most cases.
Sort a Dictionary by Value
If you do want to order a dictionary alphabetically or numerically by its values, you can do so by retrieving the values and applying the sorted function.
dictionary = {"c": 3, "a" : 1 , "d" : 4 , "b" : 2} sorted(dictionary.values()) # [1, 2, 3, 4]
The result is a list of values without the keys. But you shouldn’t need the keys anyways, because their only job in a dictionary is to provide a mapping to the values. If the values follow an order by which they can be found, the keys become obsolete.
Sort a Dictionary by Key
To order a dictionary by its keys, you can use a combination of the sorted function and a lambda expression.
dictionary = {"c": 3, "a" : 1 , "d" : 4 , "b" : 2} sort_orders = sorted(dictionary.items(), key=lambda x: x[1]) print(sort_orders) # [('a', 1), ('b', 2), ('c', 3), ('d', 4)]
This results in a list of key-value pairs.
Are Dictionaries in Python Ordered?
What if you simply want to preserve the order in which items have been added?
Python, until recently, did not preserve the order in which items have been added. But with the introduction of Python 3.6, dictionaries are ordered. To be on the safe side, I generally recommend not to rely on the assumption that dictionaries are ordered, even if you are on Python 3.6 or later. Your code will not be backward compatible with earlier versions of Python, and you probably won’t even realize it.
How To Iterate Through a Dictionary In Python?
You can either iterate through a dictionary’s keys, a dictionary’s values, or both at the same time.
dictionary = {"c": 3, "a" : 1 , "d" : 4 , "b" : 2} for key in dictionary.keys(): print(key) # c a d b for val in dictionary.values(): print(val) # 3 1 4 2 for item in dictionary.items(): print(item) # ('c', 3) # ('a', 1) # ('d', 4) # ('b', 2)
What is a Tuple in Python?
A Python tuple is an ordered collection of objects. Contrary to a list, where you can add and remove items after creation, a tuple is immutable. Tuples are useful when you need to keep two or more items together throughout operations without being altered, or removed. For example, if you want to return more than one object from a function, you could combine the objects in one tuple and have the function return this tuple. You create a tuple using parentheses or explicitly using the tuple function.
t = (1, 2, 3) t = tuple([1, 2])
The latter example we actually convert a list to a tuple. The explicit function call also allows you to convert other data structures such as dictionaries to a tuple.
t = tuple(dictionary) t # ('c', 'a', 'd', 'b')
To create an empty tuple, you use two parentheses. For a tuple with one value, you need to include an additional comma.
t = () t_1 = (1,)
Tuple elements are accessed like elements in a list.
t = (1, 2, 3) t[0] # 1
Can you Add and Delete Tuple Elements?
Since tuples are immutable you do not have the option of adding or deleting elements from an existing tuple. You can, however, concatenate two tuples to form a new one.
t1 = (1, 2, 3) t2 = (4, 5, 6) t3 = t1 + t2 t3 #(1, 2, 3, 4, 5, 6)
When it comes to deleting, you can only delete the entire tuple.
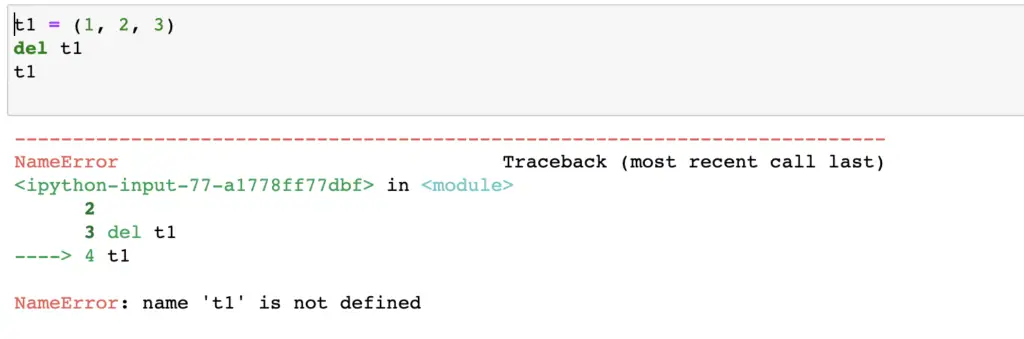
What is a Set in Python?
Sets in Python are sequences that are unordered, immutable, and do not have duplicate values.
You create a set using curly brackets or the set constructor.
s = {1,2,3,4} s = set((1,2,3,4))
Sets can contain different data types such as strings, integers, and booleans.
s = {1,"a",True, 3}
Sets are not subscriptable. Contrary to the other sequence types that we have encountered so far, you cannot access elements using a key or an index. To find a specific element, you have to iterate through the set to find it.
for l in s: print(l)
You can check whether an item is contained in a set as follows.
print(3 in s) # True
How to Add Items To a Set in Python
You can add items to a set using the add method on the set object.
s = {1,2,3,4} s.add(5) print(s) #{1, 2, 3, 4, 5}
Since items in a set have to be unique, elements that are already contained will not be added. However, you will not get an error.
s = {1,2,3,4} s.add(1) print(s) {1, 2, 3, 4}
To add another set to your existing set, Python provides the update method.
s_1 = {1,2,3,4} s_2 = {6,7,8} s_1.update(s_2) print(s_1) # {1, 2, 3, 4, 6, 7, 8}
How to Remove an Element from a Set in Python?
For removing elements from a set, Python provides the remove method.
s = {1,2,3,4} s.remove(3) s # {1, 2, 4}
How to Obtain the Set Intersection in Python
Sets in Python have an intersection method that you can use to calculate the overlapping elements between two or more sets. If you have more than two sets, the element needs to be contained in each set to be part of the intersection
s_1 = {1,2,3,4} s_2 = {2,3,8} s_3 = {2,5,8} print(s_1.intersection(s_2)) #{2, 3} print(s_1.intersection(s_2, s_3)) # {2}
How to Obtain the Set Difference in Python
The difference between two sets in Python consists of the elements that are contained in the first set but not in the second. In other words, the elements contained in both sets are not part of the difference.
To obtain the set difference, you call the difference method on the first set and pass the second set as an argument.
s_1 = {1,2,3,4} s_2 = {2,3,8} print(s_1.difference(s_2)) # {1, 4} print(s_2.difference(s_1)) # {8}
If you pass more than one set as arguments to the set method, the difference is only the elements that are contained in the first set you call the method on, but not the sets that you pass to the method call.
s_1 = {1,2,3,4} s_2 = {2,3,8} s_3 = {2,4,8} print(s_1.difference(s_2, s_3)) #{1}
How to Obtain the Set Union in Python
Finally, the union between two sets are all elements that are contained in either of the two sets including those in both. However, duplicates are not included in the union.
s_1 = {1,2,3,4} s_2 = {2,3,8} print(s_1.union(s_2)) # {1, 2, 3, 4, 8}
How to Convert a List to a Set in Python
List set conversion is done easily by calling the list function on the set.
l_1 = [1,2,3,4] s_1 = set(l_1) type(s_1)
How to Convert a Set to a List in Python
Conversely, you can convert a set to a list by calling the list function.
s_1 = {1,2,3,4} l_1 = set(s_1) type(l_1)
Converting to a set and back to a list is a convenient way to get rid of duplicates in a list.
l_1 = [1,1,2,3,4,4] s_1 = set(l_1) l_2 = list(s_1) print(l_2) #[1, 2, 3, 4]