Basic OOPs Concepts in Java
This post introduces the basics of object-oriented programming and how to create classes and objects in Java. We discuss the uses of Java keywords like, “new”, “this” or “static” as well as the access modifiers “public” and “private”.
What is a Class in Java?
A class is the elementary building block of an object-oriented program. Classes are used to create objects. A class can have attributes, and methods that define the behavior of an object.
Let’s say you have a class called a bike. A bike has certain attributes like its color and the number of gears. You define these attributes as variables. Color is defined as a piece of text such as “red”, which is why you need to precede the variable name with the String type.
“Gears” is a countable number, therefore you precede the name with the “int” type.
class Bike { String color; int gears; }
Furthermore, you can define methods to obtain those variables such as getColor and getGears as well as methods to set those variables such as setColor and setGears. Those methods are known as getters and setters in Java. They also need to explicitly include the type that these methods return.
class Bike { String color; int gears; String getColor() { return color; } int getGears() { return gears; } void setColor(String color) { this.color = color; } void setGears(int gears) { this.gears = gears; } }
In this code we have two more words that we haven’t introduced.
In the case of the setters, you are only setting the values of class variables but are not returning anything. It is a void Java method therefore you are using the “void” return type.
The little word this in Java is there to indicate that you are referring to the class variable. You are passing the variable “color” into the “setColor” method. This variable is local to the method which means it cannot be used outside the method. Then you are assigning this variable to the class variable of the same name. To explicitly distinguish the two, you precede the class variables with this to refer to this class.
Public vs Private in Java
In the previous example I left out the access control modifiers to avoid overwhelming you. Im Java you generally define explicitly who can access classes, variables and methods using access modifiers. The most common ones are “public” and “private”.
- Public: Anyone can access the code. For example if you define a method as public code in other classes, other packages, and even external code can call this method.
- Private: No one can access the code except for other code in the same class. If you define a method as private, it can only be used by other methods in the same class.
In a simple Java class like the one above, it is best practice to declare class variables as private. The rationale behind this is a principle called encapsulation. If anyone outside the class can change the value of the class variables, it can become very hard to control the behaviour of the class once the program reaches a certain size. It is much easier to end up with unintended feedback loops and the system may become non-deterministic.
Instead, you define getters and setters to change or retrieve class variables. They have one narrow purpose, which is explicitly stated in their method name. This greatly reduces the potential for unintended consequences.
So our class with access modifiers looks like this:
public class Bike { private String color; private int gears; public String getColor() { return color; } public int getGears() { return gears; } public void setColor(String color) { this.color = color; } public void setGears(int gears) { this.gears = gears; } }
How to Create Objects in Java
Once you’ve defined a class, you can create objects of that class. Previously we defined a bike class. Defining the class is like writing the specifications for how the bike should be built. Next, you actually have to build bike objects according to that specification. Programmers often say they instantiate Java classes. In sanitation of a class is done with the new keyword in Java:
// Bike my_bike = new Bike();
When you instantiate a new class you first have to define the object type of Bike, followed by the desired name of your bike object “my_bike“.
How to Call a Method in Java
In Java calling a method is simple once you have instantiated the class. If we want to retrieve the number of gears from our newly created bike, we call the getGears method on the my_bike object. Using Java’s print method, we subsequently print the number of gears.
// int gears = my_bike.getGears(); System.out.println(gears); // returns 0
To be able to instantiate our bike class and call this method, we need an entry point into our program where we can execute code. The simplest way for demonstration is to define a java main method right inside the class
public class Bike { private String color; private int gears; //... public static void main(String[] args){ Bike my_bike = new Bike(); int gears = my_bike.getGears(); System.out.println(gears); } }
In an IDE such as Intellij you should now be able to execute the code by clicking the “run” button and you should see the output in the console. Since we actually haven’t defined the number of gears, the print method will print give us the default value zero.
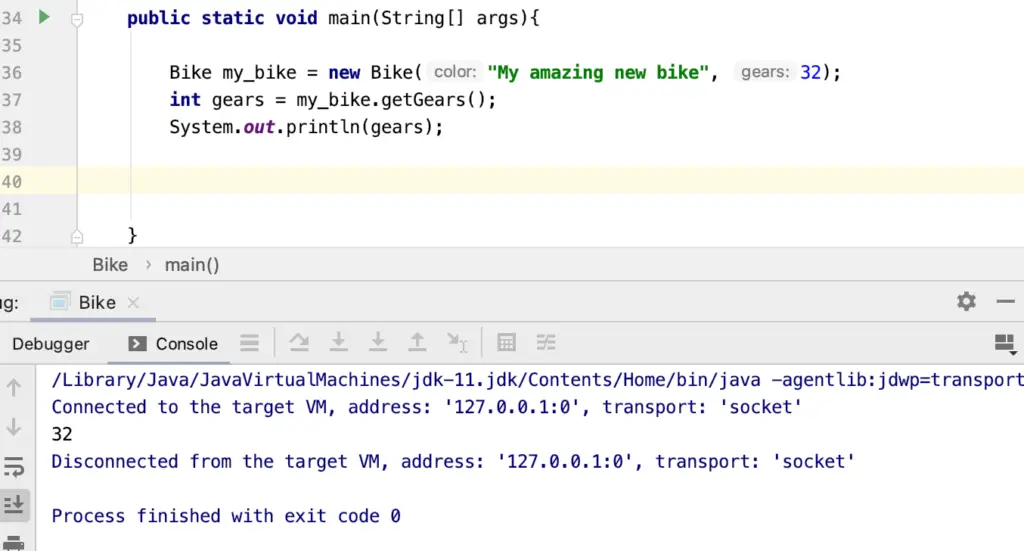
What is a Constructor in Java
To define initial variables upon class creation, we use constructors. Java constructors are a special type of method that has the same name as the class. For our Bike class, the constructor would look like this:
public class Bike { private String color; private int gears; public Bike(String color, int gears){ this.color = color; this.gears = gears; } }
If we try to instantiate the class as before, the compiler will give us an error because we haven’t supplied the necessary info to the constructor. To get the program to work, we now have to instantiate the class according to the constructor signature. A method signature in Java and other programming languages defines what arguments go into a method call.
public static void main(String[] args){ Bike my_bike = new Bike("My amazing new bike", 32); int gears = my_bike.getGears(); System.out.println(gears); }
If you don’t provide a custom constructor, the program will apply the Java default constructor
What Does Static Mean in Java?
Methods, variables and some classes can be made static in Java. If you define a static method or a static variable in Java, it means they can be accessed and used without an instance of the class they are defined in.
Let’s add a static method to retrieve the number of wheels of “Bike”. In our case, a bike generally has two wheels independent of the particular instance. Therefore, we make it a static method in the class.
public class Bike { //... public static int getNumberOfWheels(){ return 2; } //... public static void main(String[] args){ System.out.println(Bike.getNumberOfWheels()); } }
We can call the method directly on the class without instantiating a bike object.
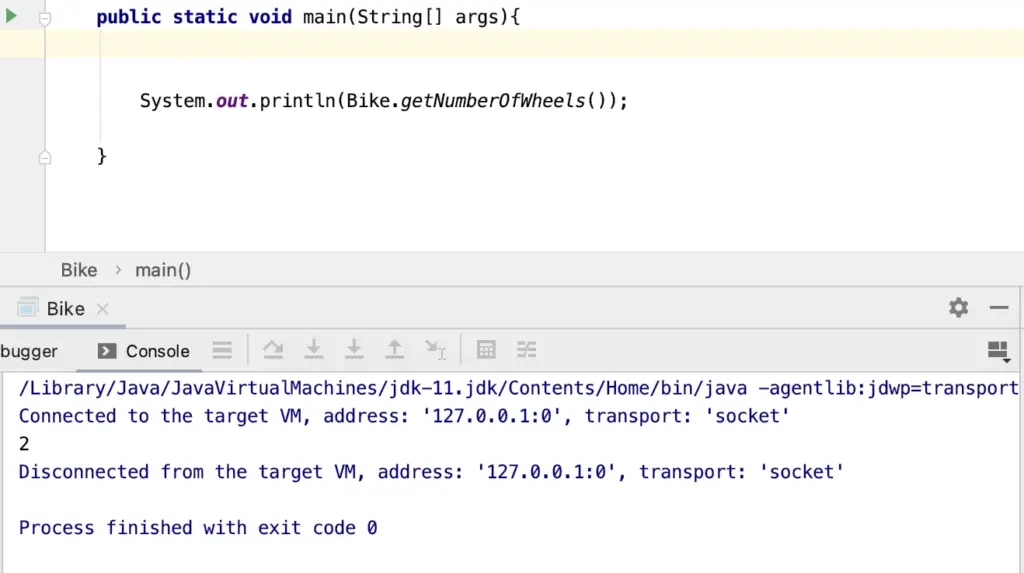
Classes are usually only defined as static when they are nested within another class. This allows us to instantiate the inner class without first instantiating the outer class. Since this is a more advanced topic, I won’t discuss it here. But if you are interested check out this post.